Thursday, May 28, 2009
OOPS with C++ Index
OOPs Concepts and Explation
Introduction of C++
Structure of C++ Program
Input output streams in C++
Cascading of input output Operators
Token of C++
Keywords in C++
Identifiers
Data Types in C++
Operators
Control structures
Constructor
Default constructor
parameterized Constructor
Constructor Overloading
Copy constructor
Destructor
Ploymorphism
Function Overloading
Operator Overloading
Inline function
Virtual function
Inheritance
Type of Inheritance
Files
class for file stream operations
Opening and closing a file
opening a File using constructor
opening files using open()
closing a file
file modes
Template
Class Template
Function Template
Exception Handling
Syntax of Exception Handling code
Review Questions
Overview Of Graphics System
Refresh Cathode Ray Tube
Raster Scan Displays
Random Scan Displays
Color Crt Monitors
Direct View Storage Tubes
Flat Panel Displays
LCD
RANDOM SCAN DISPLAY
Raster Scan Display
Color CRT Monitors
Two method to produce color Display s are
1. Beam Penetration Method
2. Shadow Mask Method
Beam Penetration Method
Random Scan Monitors
Two Layer of Phosphor – Red & Green
Speed of Electrons decides the color
Shadow Mask Method
raster scan monitors colors red , green, blue three electron gun
A Shadow Mask through which Electron Beam falls on the screen Shadow Mask CRT
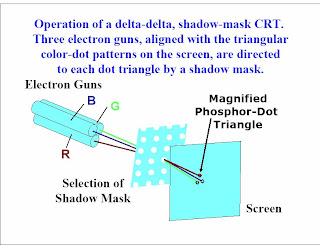
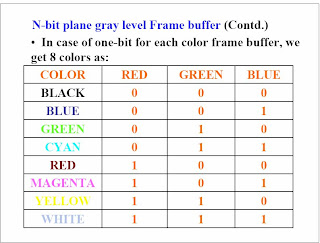
Direct View Storage Tube
Stores picture as a charge distribution just behind the phosphor coated screen
Two Electron Gun used
1. primary Gun
2. Flood Gun
Primary Gun - store picture pattern
Flood Gun - maintain picture display
Advantage – No refreshing, very high resolution without flicker,
Disadvantage - Selected parts of picture cannot be erased
FLAT PANEL DISPLAY
Reduced volume,weight ,power than crt
Two categories
emissive display(emitters)
non emissive display
Emissive display-convert electrical energy into light(Plasma Panels, Led)
Nonemissive display-convert light into graphics pattern(LCD)
Plasma panel display called as gas discharge display
Glass plates
Neon gas
Vertical & horizontal conducting ribbon
Firing voltage ,gas break down into plasma of electrons and ions
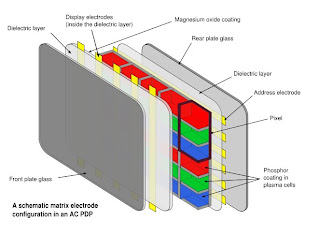
LIQUID CRYSTAL DEVICES
Calculators
Polarized light is twisted so it passes through the opposite polariser
Light then reflected back to the viewer
Picture definition in refresh buffer(60frames/sec)
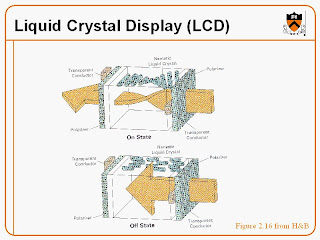
RASTER SCAN SYSTEMS
Frame buffer can be anywhere in memory
Video controller accesses the frame buffer to refresh the screen
Video controller given direct access to frame buffer memo
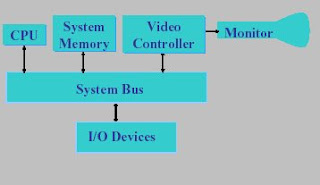
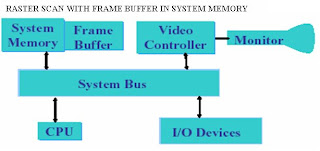
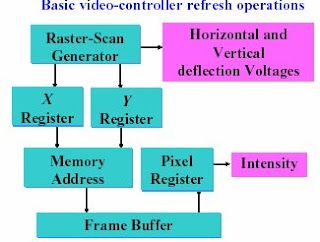
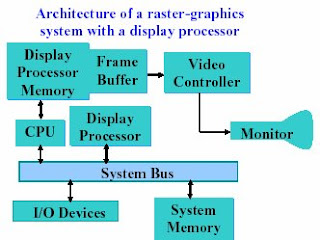
Scan conersion –a picture definition is stored in frame buffer as pixel intensity
random scan systems
Picture stored in display file stored in memory
Display processor access this display file to refresh screen
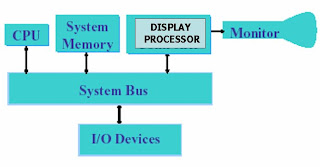
Input Devices
DDA LINE DRAWING ALGORITHM
2. dx = x2-x1
3. dy = y2-y1
4. if abs (dx) > abs (dy) |then
Step=abs(dy)
Else
Step=abs(dy)
End if
5. xin = dy / step
6. yin==dy / step
7. x = x1
8. y = y1
9. print x, y
10. for (k=1; k<=step; k++)
x = x + xin
y = y + yin
print x, y
STOP
BRENHAM’S LINE DRAWING ALGORITHM
1. Get the co-ordinates of line segment (x1, y1) and (x2, y2)
2. dx = abs(x2-x1)
3. dy = abs(y2-y1)
4. p= 2 * dy - dx
5. if x1 > x2
x = y2
y = y2
xend = x1
else
x = x1
y = y1
xend = x2
6. set pixel(x, y)
7. while x < xend
if p < 0 then
x = x + 1;
y = y;
p = p + 2 * dy
else
y = y + 1;
x = x + 1;
p = p + 2 * ( dy - dx )
8. set pixel(x, y, 1)
9. STOP
BRENHAM’S CIRCLE DRAWING ALGORITHM
2. x = xc
3. y=r
4. plot points( )
5. p = 1 - radius
6. while x < y
if p < 0 then
x = x + 1
y=y
p = p+2*x+1;
else
x = x + 1
y = y - 1;
p = p + 2 * ( x – y ) + 1
plotspoints( )
Stop
7. poltpoints( )
setpixel ( xc + x, yc + y, 1 )
setpixel ( xc +x, yc - y, 1 )
setpixel ( xc - x, yc + y, 1 )
setpixel ( xc - x, yc - y, 1 )
setpixel ( xc + y, yc + x, 1 )
setpixel ( xc + y, yc - x, 1 )
setpixel ( xc - y, yc + x, 1 )
setpixel ( xc - y, yc - x, 1 )
STOP
Line Clipping Algorithm
COHEN SUTHERLAND LINE CLIPPING ALGORITHM
1. Read the coordinates of line and draw a line
2. Read the coordinates of window xwmin, xwmax, yxmin, ywmax and draw a
window
3. Generate a vector C which determines whether the point is right, left, bottom or top
to window
4. Left if x < style=""> c[0]=1 else c[0]=0
Right if x < style=""> c[1]=1 else c[1]=0
Bottom if x < style=""> c[2]=1 else c[2]=0
Top if x < style=""> c[3]=1 else c[3]=0
5. To determine whether the entire line is inside the window Logically Or the vector
if the result is Zero the line is fully inside the window
6. To determine whether the entire line is outside the window logically AND the vectors if
result is ONE then entire line lies outside the window
1. If portion of line outside the window calculate the point where it intersects window and
suitably swapping pointsand vectors using following
Left c[0] = 1 y = y1 + ( x - x1 ) * m; x=xwmin
Right c[1] = 1 y = y2 + ( x - x2 ) * m; x=xwmax
Bottom ` c[2] = 1 x = x1 + ( y - y1 ) / m; y=xwmin
. Top c[3] = 1 x = x1 + ( y - y2 ) / m; y=xwmax
2. Draw the line using new points
3. STOP
Thursday, May 21, 2009
COMPUTER GRAPHICS -INDEX
Overview Of Graphics System
Refresh Cathode Ray Tube
RANDOM SCAN DISPLAY
Raster Scan Display
Color CRT Monitors
Direct View Storage Tube
LIQUID CRYSTAL DEVICES
RASTER SCAN SYSTEMS
Input Devices
DDA LINE DRAWING ALGORITHM
BRENHAM’S LINE DRAWING ALGORITHM
BRENHAM’S CIRCLE DRAWING ALGORITHM
CLIPPING
Question Paper December 2008
MC1652 OBJECT ORIENTED PROGRAMMING
1. What do you mean by Data Abstraction?
2. Differentiate classes and objects.
3. List out the advantages of an Inline function
4. What is the use of Dynamic allocation?
5. List out the operators that cannot be overloaded.
6. List out the operators that cannot be overloaded.
7. Distinguish a Virtual function from a Pure Virtual function.
8. What is the use of Container Classes?
9. What are the reasons for having concrete types when we can have abstract types?
10. What is the need for an Abstract class?
11. a) Explain the following programming concepts.
i) procedural Programming. (8)
ii) Modular Programming (8)
b) Explain the features of C++ Programming. (16)
12. a) Explain the following with sample application.
i) Friend function. (8)
ii) Friend classes. (8)
b) Write a program for 2 dimensional array multiplication using pointers.(16)
13. a) Write a program to overload the following operators:
i) Member Operator. (8)
ii) New and Delete Operator. (8)
b) Write a program to overload the following operator for Array.
i) Binary Operator for substraction.(4)
ii) Per increment.(4)
iii) Unary minus using friend function.(4)
iv) Unary minus.(4)
14. a) Explain the different types of Exception. How will you handle Exceptions. Explain with sample program.
b) Explain different types of inheritance with different types of access specifier.
15. a) Explai9n the role and different types of classes with an example.
b) What do you mean by Application Frameworks explain with sample application?
Review Questions
OBJECT ORIENTED PROGRAMMING
Previous Next
1.Define the terms
a) Object
b) class
2. What do you meant by an abstraction ? Explain the five different types of abstraction.
3. Explain the concept of ploymorphism and inheritance.
4. What is the structure of C++ Program
5. When do you use the keyword return and not use the keyword return?
6. What is the use of header files in C++ Program?
7. What are the new operators available in C++
8. What is function? What the steps in using a function?
9. What is the difference between inline function and ordinary function.
10. What is meant virtual and pure virtual function?
11. What is a pointer? Explain the advantages of pointers.
12. What is a class?
13. How does a class accomplish encapsulation and data hiding.
14. How do you create an object.
15. What is constructor
16. What is Destructor
17. How do we invoke a constructor and destructor function
18. What is friend function
19. What are types of constructors
20. Define ploymorphism and explain with an example.
21. What is exception handling
22. How an exception handling is defined and invoked in a program
23. Explain the applications of object oriented programming
24. Explain the basic concepts of object oriented programming in details
25. Explain Object Oriented Languages
26. Explain Software evaluation
27. What is Copy Constructor ? Explain with an example?
28. Explain function overloading with example?
29. Explain operator overloading with example?
30. Which operators can't overload?
31. When do we use the protected visibility specifier to a class member?
32. What is abstract class?
33. What is virtual function? Write a program to explain virtual function.
34. What is virtual base class?
35. Explain file operation in details with example
36. What is file mode? List of file modes
37. How do you open and close a file in C++?
38. What is template? Write a program to explain function template
39. What are the input output stream class?
40. What is class template? explain with an example
41. Explain various type of inheritance
42. What is the use of Exception Handling and Templates?
Question Paper for MCA - December 2006
DECEMBER 2006
Previous Next
MC1652 Object Oriented Programming
1.What is an abstraction?
2. State why class is user defined data type?
3. State any two ways as how inline functions speed up processing.
4. Give the syntax of the reference operator.
5. What operators can't be overloaded?
6. What is constructor overloading?
7. Can virtual functions be static? Is it possible to declare virtual function as friend for another class?
8. What is the role of throw and catch statement?
9. List out the uses of abstract type.
10. What is application framework?
11. a)Compare the paradigms procedural programming and object oriented programming. (16)
b). Explain the following object oriented features.
(i). Data abstraction
(ii). Concrete types
(iii). Abstract types and state how these features support the key features of object oriented paradigm. (16)
12. a) Write a program to declare friend function in two classes. Calculate the sum of integer data members of both the classes using friend sum() function. (16)
b). i). What are inline functions? Discuss its advantages and disadvantages.(8)
ii). What is reference? Give the syntax of the referencing operator. (8)
13. a) Write a program using function overloading to compute the sum of the matrix and the sum of the diagonal elements of the given 3 x 3 matrix. (16)
ii). Describe the rules for operator overloading. (8).
14. a) With suitable examples, explain the different froms of inheritance in details (16)
b) (i) What is a template ? Discuss about function template. (8)
(ii) Discuss the functions of keyworks tyr, catch and throw. (8)
15. a) (i) Compare the usage of concrete types and abstract type. (8)
(ii) explain the roles of classes. (8)
b) (i) discuss the uses of handles. (8)
(ii) Write short notes on actions and interface class.(8)
Question Paper for MCA Degree -December 2005
2005 December
Previous Next
MC1652 Object Oriented Programming
1. What is object oriented programming?
2. What is object based language?
3. What is dynamic allocation?
4. What are pointers?
5. Define Class
6. What is the use of constructor?
7. Distinguish between overloading and overriding.
8. What is template?
9. What is class interface?
10. Mention the major tasks in object oriented design.
11. (i) What is operator overloading? List out the rules to overload an unary operator. (6)
(ii) Write a C++ program to add two matrices using overloaded + operator. (10)
12. a) (i) Explain the characteristics of OOPs. (8)
(ii) List out the applications of OOPs.(8)
b) (i) Compare OOP and POP. (8)
(ii) Write short notes on Object oriented design. (8)
13. a) (i) Explain operators available in C++. (8)
(ii) Explain about dynamic allocation in C++. (8)
b) (i) Distinguish between a class and an object.(6)
(ii) Write a C++ program to display the given number in words for example. If the input is 123 and the output is ONE TWO THREE. (10)
14. a) (i) Explain about virtual function with an example. (8)
(ii) Distinguish between static binding and dynamic binding. (8)
b) (i) Write a C++ program to implement hybrid inheritance.(8)
(ii) Write a C++ program to create a file with set of names and read the data from the file and arrange the names in alphabetical order and store in an another file. (8)
15. a) With the help of suitable examples, explain as to how relationship among classes could be used in the efficient design of an object oriented system.
b) Briefly describe the design of an airline reservation system.
Wednesday, May 20, 2009
Arithmetic Operators
The five arithmetical operations supported by the C++ language are:
+ addition
- subtraction
* multiplication
/ division
% modulo
Operations of addition, subtraction, multiplication and division literally correspond with their respective mathematical operators.
The only one that you might not be so used to see may be modulo; whose operator is the percentage sign (%). Modulo is the operation that gives the remainder of a division of two values.
For example, if we write:
a = 10 % 3;
the variable a will contain the value 2, since 1 is the remainder from dividing 10 between 3.
Logical operators
For example
!(6 == 6) // evaluates to false because the expression at its right (6 == 6) is true.
!(8 <= 4) // evaluates to true because (8 <= 4) would be false.
!true // evaluates to false
!false // evaluates to true.
a b a&b
true true true
true false false
false true false
false false false
The operator || corresponds with Boolean logical operation OR. This operation results true if either one of its two operands is true, thus being false only when both operands are false themselves. Here are the possible results of a || b:
a b a || b
true true true
true false true
false true true
false false false
For example
( (6 == 6) && (3 > 8) ) // evaluates to false ( true && false ).
( (6 == 6) || (3 > 8) ) // evaluates to true ( true || false ).
Assignment Operators
When we want to modify the value of a variable by performing an operation on the value currently stored in that variable we can use compound assignment operators:
expression is equivalent to
value += increase; ----- value = value + increase;
a -= 5; --------- a = a - 5;
a /= b; -------- a = a / b;
price *= units + 1; -------- price = price * (units + 1);
and the same for all other operators. For example:
compound assignment operators in
#include "iostream"
using namespace std;
int main ()
{
int a, b=3;
a = b;
a+=2; // equivalent to a=a+2
cout <<>
return 0;
}
Increment and decrement Operators
c++;
c+=1;
c=c+1;
are all equivalent in its functionality: the three of them increase by one the value of c.
In the case that the increase operator is used as a prefix (++a) the value is increased before the result of the expression is evaluated and therefore the increased value is considered in the outer expression; in case that it is used as a suffix (a++) the value stored in a is increased after being evaluated and therefore the value stored before the increase operation is evaluated in the outer expression. Notice the difference:
Example 1
B=5;
A=++B; // A contains 6, B contains 7 B=5;
Example 2
B=5;
A=B++; // A contains 5, B contains 6
!= Not equal to
> Greater than
<>
>= Greater than or equal to
<= Less than or equal to
Here there are some examples:
(7 == 5) // evaluates to false.
(5 > 4) // evaluates to true.
(3 != 2) // evaluates to true.
(6 >= 6) // evaluates to true.
(5 <>
Of course, instead of using only numeric constants, we can use any valid expression, including variables. Suppose that a=2, b=3 and c=6,
(a == 5) // evaluates to false since a is not equal to 5.
(a*b >= c) // evaluates to true since (2*3 >= 6) is true.
(b+4 > a*c) // evaluates to false since (3+4 > 2*6) is false.
((b=2) == a) // evaluates to true.
Conditional Operators
logical-or-expression
logical-or-expression ?
expression : conditional-expression
The conditional operator (? :) is a ternary operator (it takes three operands).
The conditional operator works as follows:
The first operand is implicitly converted to bool. It is evaluated and all side effects are completed before continuing.
If the first operand evaluates to true (1), the second operand is evaluated.
If the first operand evaluates to false (0), the third operand is evaluated
The result of the conditional operator is the result of whichever operand is evaluated — the second or the third. Only one of the last two operands is evaluated in a conditional expression.
Conditional expressions have right-to-left associativity. The first operand must be of integral or pointer type.
The following rules apply to the second and third expressions:
If both expressions are of the same type, the result is of that type.
If both expressions are of arithmetic or enumeration types, the usual arithmetic conversions (covered in Arithmetic Conversions) are performed to convert them to a common type.
If both expressions are of type void, the common type is type void.
If both expressions are of a given class type, the common type is that class type.
Any combinations of second and third operands not in the preceding list are illegal. The type of the result is the common type, and it is an l-value if both the second and third operands are of the same type and both are l-values.
Example
#include
using namespace std;
int main()
{
int i = 1, j = 2;
cout << ( i > j ? i : j ) << " is greater." <<>
}
Bitwise Operators
The bitwise operators are:
Bitwise AND (&)
Bitwise exclusive OR (^)
Bitwise inclusive OR (|)
These operators return bitwise combinations of their operands.
and-expression
equality-expression
and-expression & equality-expression
Operator Keyword for &
Example
#include
using namespace std;
int main()
{
unsigned short a = 0xFFFF; // pattern 1111 ...
unsigned short b = 0xAAAA; // pattern 1010 ...
cout <<>
}
exclusive-or-expression
and-expression
exclusive-or-expression ^ and-expression
The bitwise exclusive OR operator (^) compares each bit of its first operand to the corresponding bit of its second operand. If one bit is 0 and the other bit is 1, the corresponding result bit is set to 1. Otherwise, the corresponding result bit is set to 0.
Both operands to the bitwise exclusive OR operator must be of integral types. The usual arithmetic conversions covered in Arithmetic Conversions are applied to the operands.
Operator Keyword for ^
The xor operator is the text equivalent of ^. There are two ways to access the xor operator in your programs: include the header file iso646.h, or compile with the /Za (Disable language extensions) compiler option.
Example:
#include
using namespace std;
int main()
{
unsigned short a = 0x5555; // pattern 0101 ...
unsigned short b = 0xFFFF; // pattern 1111 ...
cout <<>
}
Bitwise Inclusive OR Operator
inclusive-or-expression
exclusive-or-expression
inclusive-or-expression | exclusive-or-expression
Operator Keyword for |
Example
#include
using namespace std;
int main()
{
unsigned short a = 0x5555; // pattern 0101 ...
unsigned short b = 0xAAAA; // pattern 1010 ...
cout <<>
}
Special Operators
Implemented new operators in C++
insertion operator <<
extraction operator >>
scop resolution operator ::
pointer-to-member decelerator ::*
pointer-to-member operator ->* .*
Memory release operator delete
Line feed Operator endl
Memoery allocation operator new
Field width operator setw
Inline function
The general form is:
Inline is a keyword prefixed with the function definition.
inline returntype functionname ( argument_list )
{
Body of the function
}
Example:
#include "iostream.h"
#include "conio.h"
inline int big(int a,int b,int c)
{
if((a>c)&&(a>b))
return (a);
else if((b>a)&&(b>c))
return (b);
else if((c>a)&&(c>b))
return (c);
else
return (0);
}
void main()
{
int x,y,z,r;
char ch;
clrscr();
lable:
cout<<"\n\n\n\n\t ENTER THE VALUES FOR X,Y AND Z :\t";
cin>> x>> y>> z;
r=big(x,y,z);
if(r!=0)
cout<<"\n\n\n\t\t BIGGEST NUMBERS IS:\t"<
else
cout<<"\n\n\t\t SAME VALUES ARE REPEATED ";
cout<<"\n\n\t DO YOU WANT TO CONTINUE[Y/N]:\t";
cin>> ch;
if((ch=='y')||(ch=='Y'))
goto lable;
getch();
}
Output:
ENTER X ,Y AND Z VALUE = 8 9 14
BIGGEST NUMBER IS: 14
Virtual function
general form is:
{
---------
---------
Virtual returntype functionname( argrument_list)
{
--------------------
--------------------
}
};
Class derived : public baseclass
{
---------
---------
returntype functionname( argument_list)
{
-----------------------
-----------------------
}
};
Accessing the virtual function:
baseclass *ptr, obj1;
derivedclass obj2;
ptr = &obj1;
ptr->functionname(arguments);
ptr = &obj2;
ptr->functionname(arguments);
#include "iostream.h"
class figure
{
protected:
double x, y;
public:
void setdim(double i, double j)
{
x = i;
y = j;
}
virtual void showarea( )
{
cout << "No area computation defined "; cout << "for this class.\n"; } } ; class triangle : public figure { public: void showarea( ) { cout << "Triangle with height "; cout << p =" &t;">setdim(10.0, 5.0);
p->showarea();
p = &s;
p->setdim(10.0, 5.0);
p->showarea();
return 0;
}
Unconditional Statements
Transferring control from one place to another place with out checking any condition is called unconditional statement.
They are:
goto statement
break statement
continue statement
The goto Statement
A goto statements can cause program control to end up almost anywhere in the program unconditionally.
The got statement requires a label to identify the place to move the execution. A label is valid variable name and must be ended with colom.
SYNTAX:
goto label;
. . . . . . . .
. . . . . . . .
label: statements;
EXAMPLE:
goto t;
cout<<” This is goto statement”;
t: cout<<”Good Morning”;
The break Statement
The break statement is used to terminate the loop. When the keyword break is used inside any ‘C’ loop, control automatically transferred to the first statement after the loop. A break is usually associated with an if statement.
SYNTAX:
break;
Example for break statement
void main ()
{
int num;
for (num=1; num<=10; num++)
{
cout <<>
if (num==6)
{
cout << "count aborted";
break;
}
}
}
Output will be
1,2,3,4,5,6 count aborted
The continue Statement
In some situations, we want to take the control to the beginning of the loop, b’ passing the statement inside the loop which have not yet been executed. For this purpose the continue is used. When the statement continue is encountered inside any ‘C’ loop control automatically passes to the beginning of the loop.
SYNTAX:
continue;
Like break statement the continue statements also associated with “if” statement.
Example for Continue Statements
void main ()
{
int num;
for (int num=10; num>0; num--)
{
if (num ==5) continue;
cout <<>
}
cout << "good";
}
Output will be:
10, 9, 8, 7, 6, 4, 3, 2, 1, good
Difference between Break and Continue Statements.
Break Statements
Break statement takes the control
to the outside of the loop
It us also used up switch statement
Always associated with if condition in loops
Continue Statements
Continue statement takes the control to the beginning of the loop
This can be used up loop statement
This is also associated with if condition.
Tuesday, May 12, 2009
CONTROL STRUCTURES
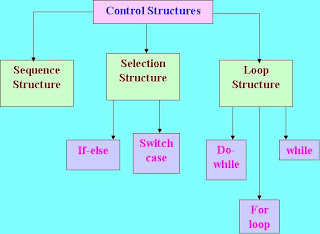
A program is usually not limited to a linear sequence of instructions. During its process it may bifurcate, repeat code or take decisions. For that purpose, C++ provides control structures that serve to specify what has to be done by our program, when and under which circumstances.
With the introduction of control structures we are going to have to introduce a new concept: the compound-statement or block. A block is a group of statements which are separated by semicolons (;) like all C++ statements, but grouped together in a block enclosed in braces: { }:
{ statement1; statement2; statement3; }
Most of the control structures that we will see in this section require a generic statement as part of its syntax. A statement can be either a simple statement (a simple instruction ending with a semicolon) or a compound statement (several instructions grouped in a block), like the one just described. In the case that we want the statement to be a simple statement, we do not need to enclose it in braces ({}). But in the case that we want the statement to be a compound statement it must be enclosed between braces ({}), forming a block.
Conditional Statements
Unconditional Statements